Command-line options parserThis class is a basic class used to parse program options. Input format requirements: More...
Classes | |
struct | OptionDesc |
Public Member Functions | |
void | setGlobalDesc (const std::string &desc) |
Add global description to the list of options. More... | |
void | addSimpleOption (const std::string &name, const OptionDesc &optionDesc) |
Add a simple option to the internal dictionary. More... | |
template<typename T > | |
void | addValueOption (const std::string &name, const OptionDesc &optionDesc) |
Add a value option to the internal dictionary. More... | |
bool | parse (int argc, char **argv) |
Parse command line options. More... | |
bool | hasOption (const std::string &name) const |
Check if an option was parsed or not. More... | |
template<typename T > | |
T | getOptionValue (const std::string &name) const |
Get option value. More... | |
void | printOptionsDesc () const |
print all option descriptions | |
Detailed Description
template<typename First, typename... Types>
class OpenViBE::ProgramOptions< First, Types >
Command-line options parser
This class is a basic class used to parse program options. Input format requirements:
- Date
- 2016-01-25
- Option prefix must be '-' or '–' e.g. cmd -help or –help
- Option value assignment must be set with '=': e.g. cmd -config=myfile.txt
- Option value that consists of pair are set with (): e.g. cmd -newToken=(key,value)
Parsing options occurs in 2 steps:- Populating the list of possible options with simple options (e.g. –help) and value options (e.g. –option=value)
- Parsing options from command line
- Todo:
- The parser has only be tested for the player use. It needs more in-depth testing to be used in another context. Moreover, it should be extended to accept any type.
- Note
- The implementation is trivial. Prefer the use of robust and fully featured boost program_options compiled library if possible.
Member Function Documentation
void setGlobalDesc | ( | const std::string & | desc | ) |
Add global description to the list of options.
- Parameters
-
[in] desc the global description in printable format
The global description is used as additional printable documentation when printOptionsDesc() is called.
void addSimpleOption | ( | const std::string & | name, |
const OptionDesc & | optionDesc | ||
) |
Add a simple option to the internal dictionary.
- Parameters
-
[in] name the option name [in] optionDesc the option description
Simple options are option withou value (e.g. –help or –version)
void addValueOption | ( | const std::string & | name, |
const OptionDesc & | optionDesc | ||
) |
Add a value option to the internal dictionary.
- Parameters
-
[in] name the option name [in] optionDesc the option description
Template paramter T: The type of the option to be added
bool parse | ( | int | argc, |
char ** | argv | ||
) |
Parse command line options.
- Precondition
- addSimpleOption and addValueOption must be called to populate options dictionary
- Parameters
-
[in] argc number of arguments [in] argv pointer to the list of arguments
- Returns
- : false if an error occurred during parsing, true otherwise
bool hasOption | ( | const std::string & | name | ) | const |
Check if an option was parsed or not.
- Precondition
- Must be called after parse()
- Parameters
-
[in] name the option name
- Returns
- : true if the option was parsed, false otherwise
Referenced by CommandLineOptionParser::parse().
T getOptionValue | ( | const std::string & | name | ) | const |
Get option value.
- Precondition
- hasOption() should be called to ensure the option is available
- Parameters
-
[in] name the option name
- Returns
- the option value (will be the default value if the option was not parsed)
Template paramter T: the type of the option to retrieve (must match the type used to set the option with addValueOption())
Referenced by CommandLineOptionParser::parse().
Generated on Tue Jun 26 2012 15:25:54 for Documentation by
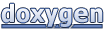